In Part 7 in the Soaring Through the Cloud series Oracle ACE Director Lonneke Dikmans focuses on Oracle Mobile Cloud Service and the role it played in the demo described in the series, providing rich client APIs for the JET application that displays proposed acts and voting results, tracking API usage, and enforcing security constraints.
By Lonneke Dikmans 
Table of Contents
- Mobile Cloud Service
In this, the seventh installment of Soaring Through the Clouds, we address Oracle Mobile Cloud Service (MCS). MCS helps organizations manage APIs they use for mobile applications and web applications based on REST/JSON. MCS sits in front of enterprise applications and mobile or web applications. The figure below shows this architecture:
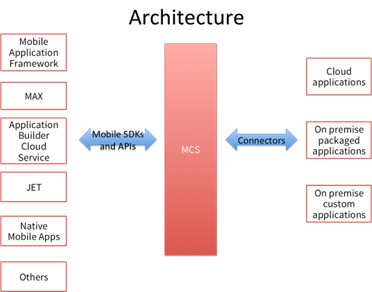
MCS was used in the demo to offer rich client APIs for the JET application that shows all proposed acts and the result of the voting. Apart from that, it was used to track usage of the APIs and enforce security constraints. The MCS APIs are backed by the SOAP Web Services implemented on SOA CS to publish the information on the current artist proposals.
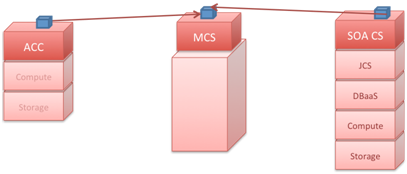
In our demo, as in real life, the developer of the user application was working separately from the developer of the backend services. To cater for this, I created contract-first APIs in MCS. These APIs provide mock data to enable the front-end developer to get started without waiting for the end-to-end integration to be finished. After that, we implemented the API by connecting it to the SOAP services, we tested the API and, finally, we published it.
Design the API
APIs, connectors and users are grouped together in MCS in a "mobile backend"; this is a convenient way to group and reuse APIs and connectors that already exist in your mobile environment and to manage the security.
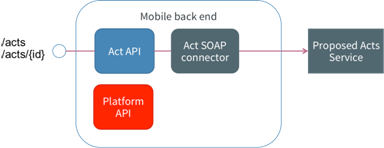
In this case, we need two REST endpoints: one to fetch all acts, and one to get the details of a specific act.
The first step is to create the API. You can do this from scratch in MCS, or you can upload a RAML file that contains a predefined API. In this case, we created the API from scratch.
After defining the name, you have to define the endpoints.
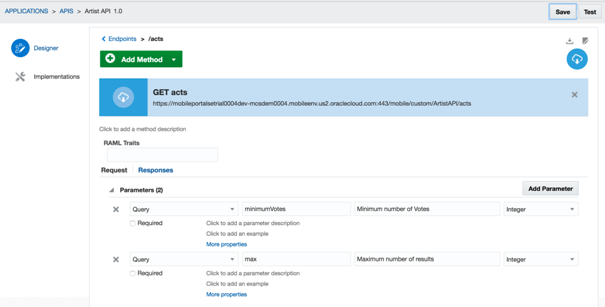
Apart from defining the parameters and resource path of the endpoints, MCS also offers the opportunity to define the response. This is very useful for the developer of the JET application: as soon as the API is added to a mobile backend, the developer can start calling the API and work with the mock response from MCS.
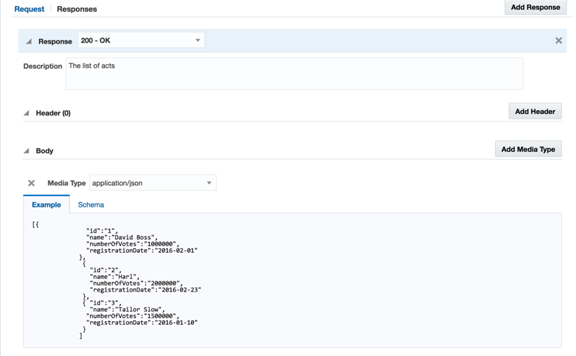
After defining the endpoints, I defined the security features of the API. You can define a role for the entire API or you can define roles per endpoint. In this case, we allowed anonymous access.
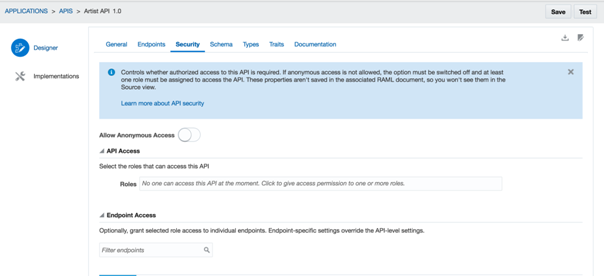
The API definitions are all stored in a RAML. Part of the resulting RAML is shown below (the entire file can be downloaded from the code repository on GitHub: https://github.com/lucasjellema/aced-cloud-demo-ofmw2016-valencia).
#%RAML 0.8
title: Artist API
version: 1.0
baseUri: /mobile/custom/artistapi
protocols: [HTTPS]
mediaType: "application/json"
/acts: displayName: Acts
description: | List of all candidate acts (with per act the name, number of votes, date added)
get:
protocols: [HTTPS]
queryParameters:
minimumVotes:
displayName: minimum number of votes
description: | minimum number of votes that an act has to have received to appear in the list
type: string
addedSince:
displayName: Added since
description: | acts that have been added since this date type: date
max:
displayName: Maximum number of proposals
description: | maximum number of acts that is returned type: integer
responses:
200:
body: application/json:
example: | [{ "id":"1",
"name":"Bruce Newjersey", "numberOfVotes":"1000000",
Calling the API
To work with the API outside MCS, the API must be associated with a mobile backend. Once that is finished, the JET developer can work with the API from within the mobile application.
Every call to the API needs the mobile backend id and the anonymous key associated with it. A call with postman to fetch all the acts looks like this:
GET /mobile/custom/artistapi/acts?minimumVotes=1&max=100 HTTP/1.1
Host: mobileportalsetrial1304dev-mcsdem0001.mobileenv.us2.oraclecloud.com:443
oracle-mobile-backend-id: 55bc25a9-52ee-4a63-8db2-ce18cab1948b
Authorization: basic TUNTREVNMDAwMV9NT0JJTEVQT1JUQUxTRVRSSUFMMTMwNERFVl9NT0JJTEVfQU5PTllNT1VTX0FQUElEOmR5Nm91NW5wX3RnbE5r
Cache-Control: no-cache
Postman-Token: 1192816b-ef7b-0e25-13dc-5f1f18064775
When called, MCS will return the mock responses defined in the RAML because the implementation of the API is set to MockService 1.0, as can be seen in the image below:
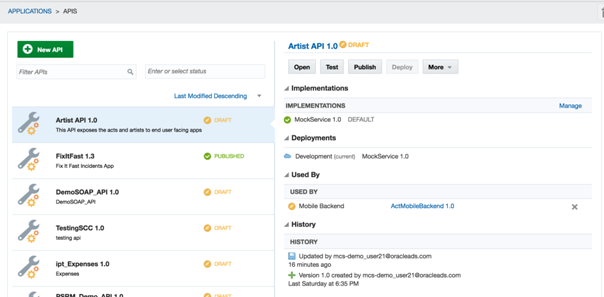
Develop the API
When a call to the API is made, data must be fetched from the backend systems. In this case, it is very simple: every API endpoint corresponds with an operation in the SOAP service. Of course this is not always the case; sometimes you need to do more elaborate work to implement the API.
In this case, implementing an API has three steps:
- Define the connector to the SOAP service
- Create a node.js application that receives the requests, calls the SOAP service and returns the response
- Upload the node.js application to MCS
Define the connector
The connector can be defined in MCS using the wizard. When you create a new connector, you can choose one of three types: REST, SOAP or ICS. In this case we chose the SOAP connector.
The SOAP connector requires you to point to a WSDL. After parsing the WSDL, the connector gives you the opportunity to define the port types and security features. Once that is done, you can test the connector and associate it with the mobile backend.
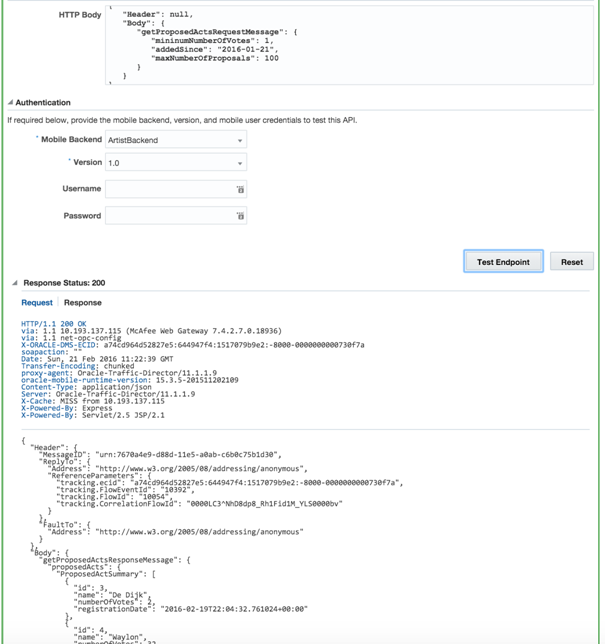
Now we have all the parts we need to create the implementation.
Writing the Node.js application
Unfortunately, you can’t write the code for the mobile backend in MCS. I used NetBeans to write the node.js application. The implementation zip file must consist of:
- A root directory that has the name of the custom code module
- A package.json file in the root directory
- Javascript files (at least one) containing the implementation
- A node_modules directory containing any additional modules required
In our case, the package.json file looked like this:
{ "name" : "artistapi",
"version" : "1.0.0",
"description" : "the API to get artist and attendance", "main" : "artistapi.js",
"oracleMobile" : {
"dependencies" : {
"apis" : { },
"connectors" : {"/mobile/connector/ActsSOAP": "1.0"}
}
}
}
Fortunately, you don’t have to write this file manually; it is part of the Javascript Scaffold file you can download for your API to get you started with the implementation.
Next, you write the code. Here’s a snippet of the code we used in the demo below:
var transform = require("./actTransformations");
-
exports.getActs = function (req, res) {
var handler = function (error, response, body) {
**console.log('body: ', body);**
var responseMessage = body;
if (error) {
res.status(500).send(error.message);
} else if (parseInt(response.statusCode) === 200) {
var json = JSON.parse(body);
var resultArray = json.Body.getProposedActsResponseMessage.proposedActs.ProposedActSummary; removeNullAttrs(resultArray);
var transformFunction = transform.actSummarySOAP2REST;
var acts = resultArray.map(transformFunction); responseMessage = JSON.stringify(acts); res.status(200).send(responseMessage);
}
res.end();
};
var optionsList = {uri: '/mobile/connector/ActsSOAP/getProposedActs'}; optionsList.headers = {'content-type': 'application/json;charset=UTF-8'}; var minimumVotes = req.query.minimumVotes ? req.query.minimumVotes : 0; var outgoingMessage = {
Header: null,
Body: {"getProposedActsRequestMessage": { "mininumNumberOfVotes": minimumVotes,
"addedSince": req.query.addedSince, "maxNumberOfProposals": req.query.max
}}};
- optionsList.body = JSON.stringify(outgoingMessage);
- console.log('optionsList: ', optionsList);
var r = req.oracleMobile.rest.post(optionsList, handler);
};
There are three things worth noting in the code:
- As you can see, there are some log statements in the code here. This is useful when you are still in development mode. The log statements show up in the administration console of the mobile backend in MCS.
- MCS has built-in functionality to transform XML SOAP messages to JSON; in our custom function, we map the fields of the response to our own AI definition.
- The uri of the connector is passed to the "post" command; the endpoint of the SOAP service is defined in the connector in MCS, separate from our code. This means that if the location of the SOAP service changes, we don’t have to change the code, we only have to edit the connector data.
Upload the node.js application
The last step is to upload the node.js application to MCS and make this, not the MockService, the default implementation. As soon as this is done, the responses that are returned will be determined by the node.js application code, not the responses defined in the RAML.
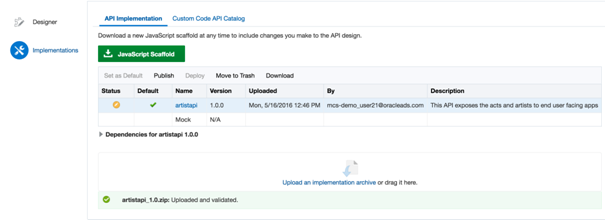
Test the API
After writing the code, I started testing it. For this purpose I have defined a Postman collection with the various calls. The result of the calls can be checked by defining tests in Postman. The log statements show up in the administration console in MCS (see Monitoring," below).
Publish the API and other artifacts
Once you are satisfied with the result, you can publish the API and deploy it to production. Once an API is published, you can’t change it anymore. Changes require that you create a new version.
To publish this application, I executed the following steps:
- Remove the log statements from the code and publish the API implementation
- Publish the API and deploy it to production
- Publish the connector and deploy it to production
- Publish the mobile backend and deploy it to production
When deploying the mobile backend, all the depending artifacts (API, implementation and connectors) must be deployed in that environment as well.
Note: As soon as you publish the mobile backend, the mobile backend id changes. The same is true when you deploy it to another environment. This means that the JET application needs to be able to change these properties, depending on what version (e.g., the draft version in development or the published version in production) it is connecting to.
Monitoring
One of the key features of MCS is the monitoring capability. As an administrator you can monitor the health of the different mobile backends, in terms both of performance and of errors. During development, I used this feature to debug mistakes I made in the mapping of the fields from the response to my API definition. During production, administrators use this feature to monitor the errors, frequency of use and performance of the APIs and mobile backends. As you can see below, there is also a "Logs" tab in the console. This is where you can find the log statements you have put in your code.
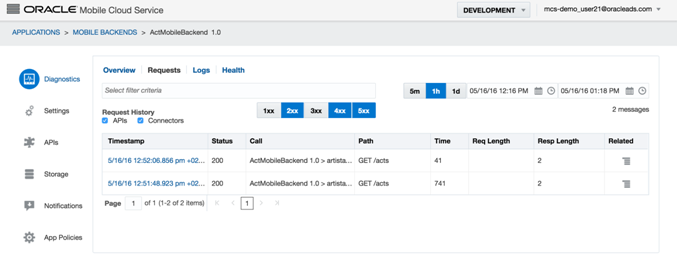
Conclusion
A number of other MCS features have not been discussed in this article because we did not use these features in our demo of the cloud services. MCS was used here to offer APIs to a web application. One could argue that we could also have used ICS for this purpose.
The added value of MCS comes with the use of:
-
Native mobile applications. MCS offers an SDK for mobile development, platform APIs like push notifications and storage, and the tracking and tracing of usage in native mobile applications (analytics).
-
Large number of APIs with different applications. Mobile backends can be associated to one or more specific mobile applications by registering the mobile app with the mobile backend. This enables you to keep track of the usage by different mobile applications, and to make sure APIs and connectors are reused in a controlled way.
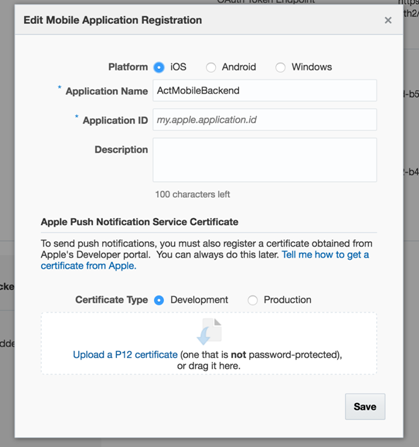
For our demonstration of cloud integration, MCS proved to be a valuable asset because of the decoupling of the front-end API from the backend API, the features to easily transform backend services to the desired mobile API, and the monitoring capabilities of the cloud service. However, the real strength of the platform would become apparent if we had shown a demonstration with a native mobile application like MAF.
About the Author
Oracle ACE Director Lonneke Dikmans is managing partner at eProseed NL and works as an architect, both on projects and as an enterprise architect. Lonneke specializes in Oracle Fusion Middleware and is a BPMN certified professional. Oracle Magazine awarded her the title of Oracle Fusion Middleware Developer of theY ear in 2007. She publishes frequently online and shares her knowledge at conferences and other community events. Lonneke is the co-author of of SOA Made Simple (2012, Packt Publishing).
NOTE: This article represents the expertise, findings, and opinion of the author. It has been published by Oracle in this space as part of a larger effort to encourage the exchange of such information within this Community, and to promote evaluation and commentary by peers. This article has not been reviewed by the relevant Oracle product team for compliance with Oracle's standards and practices, and its publication should not be interpreted as an endorsement by Oracle of the statements expressed therein.