While there are many JavaScript frameworks and libraries to choose from, few cover all the typical requirements for enterprise applications. This article by Oracle ACE Director Andreas Koop examines Oracle JET’s potential as an enterprise-grade framework for end-to-end client-side web application development, and then shows you how to get started building your first JET application.
by Andreas Koop 
It was one of the great announcements at Open World 2015: Oracle JET, the JavaScript Extension Toolkit, entered the stage of client-side web application development frameworks. Although a lot of JavaScript frameworks and libraries are out there, very few cover all requirements that typically exist for enterprise applications. Oracle JET promises to be an enterprise-grade framework for end-to-end client-side web application development. In this technical article, I am going to give an overview of Oracle JET, its main concepts and how you can get started to build your first JET application.
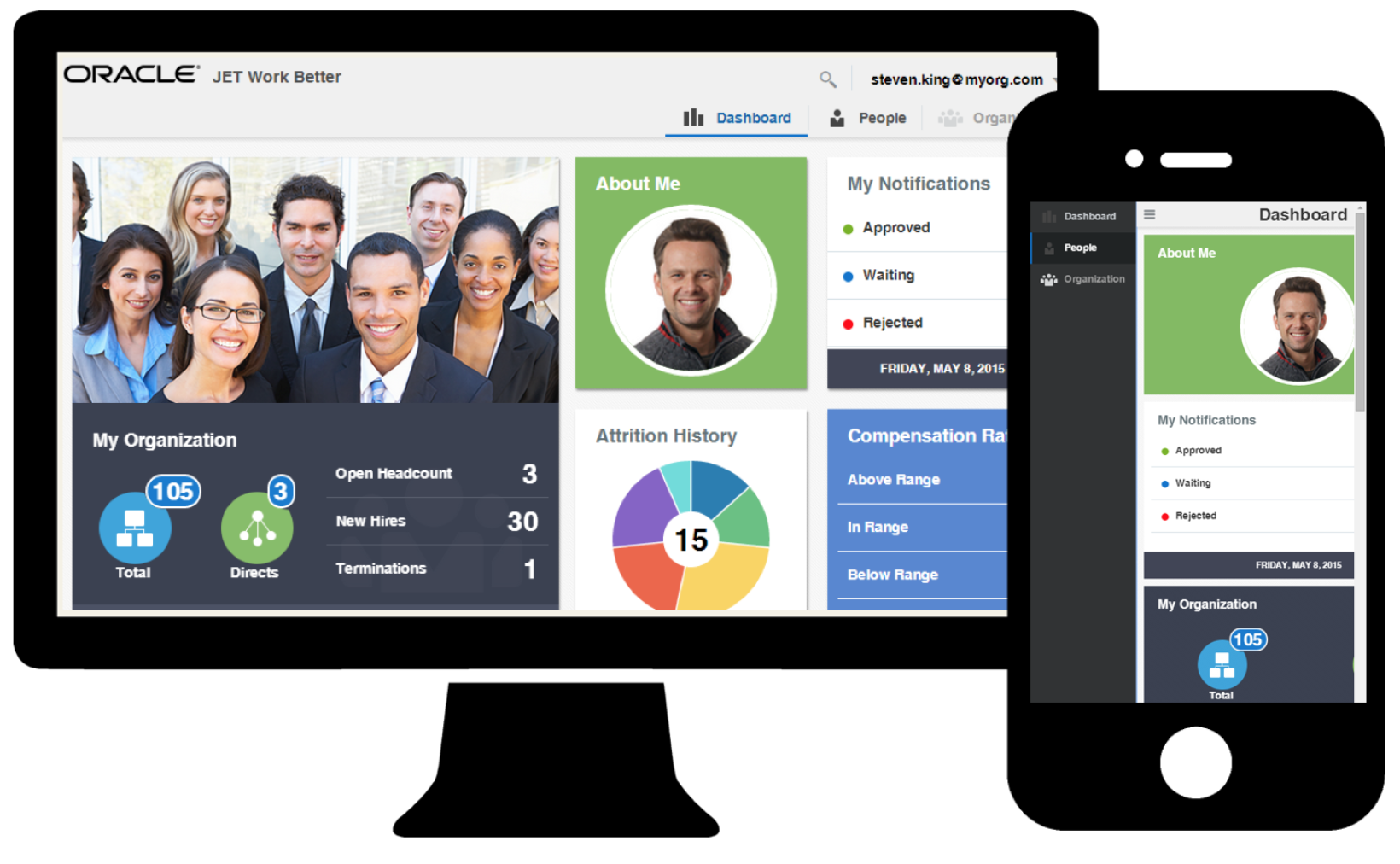
Architecture for Modern Web Applications
Before doing a deep dive into implementation details, let's look at web architectures and patterns in order to classify Oracle JET properly and get a better understanding. The de facto standard pattern is MVC (Model-View-Controller) on the server side. Whether you are using Perl, PHP, Phyton or the Oracle/Java-based techniques like JSPs (Java Server Pages), Struts (Open Source Apache Framework), JSF (Java Server Faces) or ADF Faces, best practice is to separate your code into Model, View and Controller. See Figure 1 for illustration of this traditional pattern.
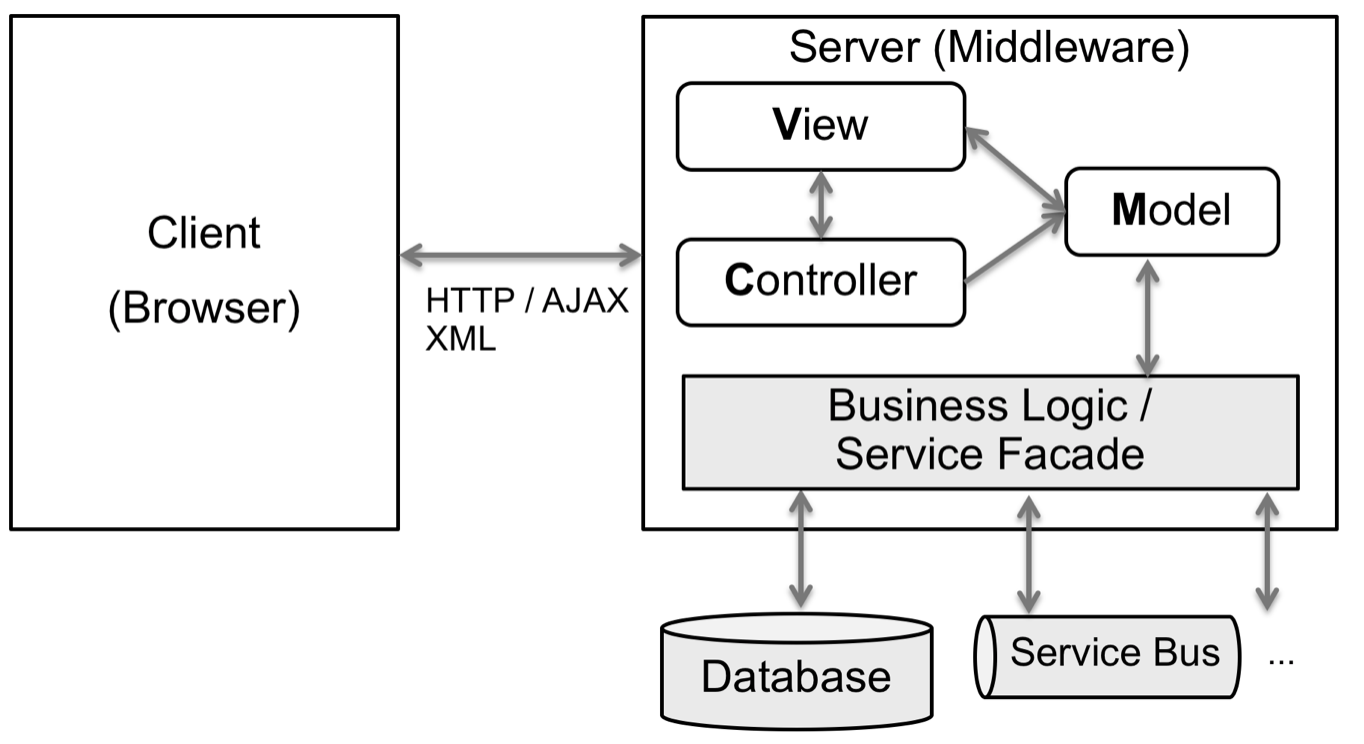
Figure 1: Server-Side Web Architecture using Model-View-Controller pattern
With the rise of HTML5, CSS3, and performant JavaScript engines, more and more is implemented on the client side. Starting with AJAX Calls and simple DOM manipulation (remember DHTML?), nowadays almost all UI logic, View Templates plus View Model is handled on the client side using JSON-based RESTful services, WebSockets or Server Sent Events (SSE) for backend communication. Business demands to target users on any device (TVs, tablets, mobiles, watches, glasses, and whatever is coming next) using Cloud Services, and the standardization of HTML5/CSS3/JavaScript, are the key drivers of the evolution of a new web application architecture for single-page applications (SPA). See Figure 2 for client-side architecture using Model-View-ViewModel pattern (MVVM).
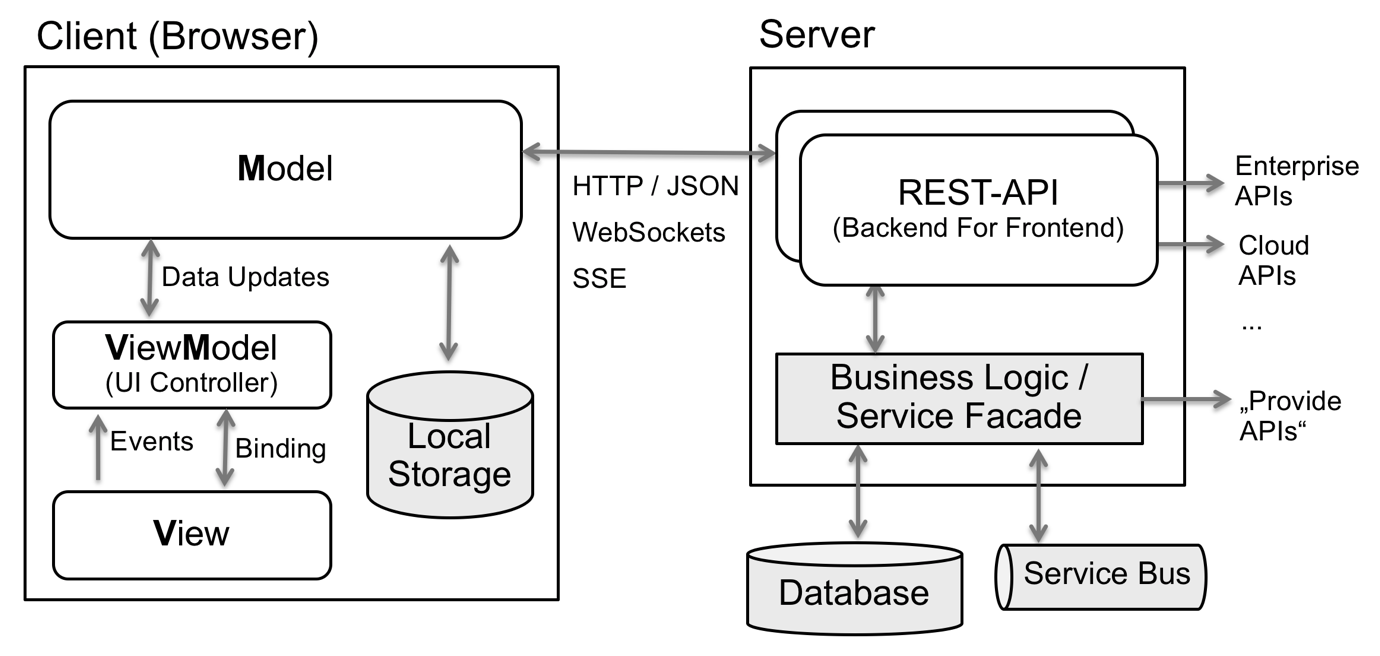
Figure 2: Client-side web architecture for SPAs using MVVM pattern
The Model represents the application data, while the ViewModel exposes data from the Model to the View and maintains the application's (UI) state. The View defines the representation of how it should be displayed visually. To improve user experience (UX), modern web applications use the local storage HTML5 feature implemented by all modern browsers.
These kinds of applications typically communicate with the backend system through JSON-based RESTful APIs. Recent evolutions recommend a dedicated "Backend For Frontend"-API (BFF-API) on the server-side which exposes application optimized APIs rather than generic enterprise APIs. The BFF-API is based on custom business logic or simply uses existing enterprise APIs or third-party Cloud APIs (e.g., Google Maps, etc).
By the way: Mobile Cloud Service (MCS) is built with exactly the same architectural principles in mind. It enables you to create app-specific Mobile Backend as a Service (MBaaS) that can be composed of existing general purpose APIs.
Oracle JET (JavaScript Extension Framework): What’s in There
Oracle JET is engineered from a group of well known and proven Open Source JavaScript libraries and Oracle-specific implementation of UI components and CSS to fully meet/implement the Alta UI Design Guides and best practices.
Alta UI is the default
From my perspective, Oracle's Alta UI initiative is a logical move. Not only does it simplify the UI and improve the overall UX, it also enables existing enterprise applications to be modernized as lean architectures using Microservices and integration through RESTful services. Unsurprisingly, the default theme for Oracle JET UI components is Alta UI.
Oracle JET Development: The Basics
JavaScript
Beyond the basic technologies--HTML(5) and CSS(3)--JavaScript is essential; without it, it will be difficult to get started.
The most important things you should know is that JavaScript is a dynamically typed programming language and that it is prototype-based. No classes exist, just objects containing attributes and functions. Functions are first-class citizens that can be used as parameters. Inheritance relations are implemented by object cloning. Attributes and Functions can easily be added at runtime. That means it is very flexible and powerful, but your code might become fragile; most errors you will notice only at runtime.
jQuery/jQuery UI
jQuery is a JavaScript library designed for HTML Document Object Model (DOM) traversal and manipulation, events, and easy AJAX handling. Since browsers interpret JavaScript slightly differently, jQuery includes an API that makes it very convenient to write clean code. All the browser specifics are encapsulated and hidden from the web developer.
jQuery UI is a set of UI widgets, interaction effects, and themes built on top of the jQuery library. Oracle JET uses these concepts to expose all JET UI components as theme-able UI widgets.
Knockout
Knockout is a lightweight JavaScript library that helps to implement the MVVM pattern for HTML5/JavaScript UIs. Its key strength is in binding DOM elements with the view model, and in implementing view templates. Any time the model changes (depending on user action or external data changes), Knockout keeps the corresponding parts in sync. See Listing 1, below, to get an idea of how Knockout binds HTML DOM elements to a JavaScript-based view model.
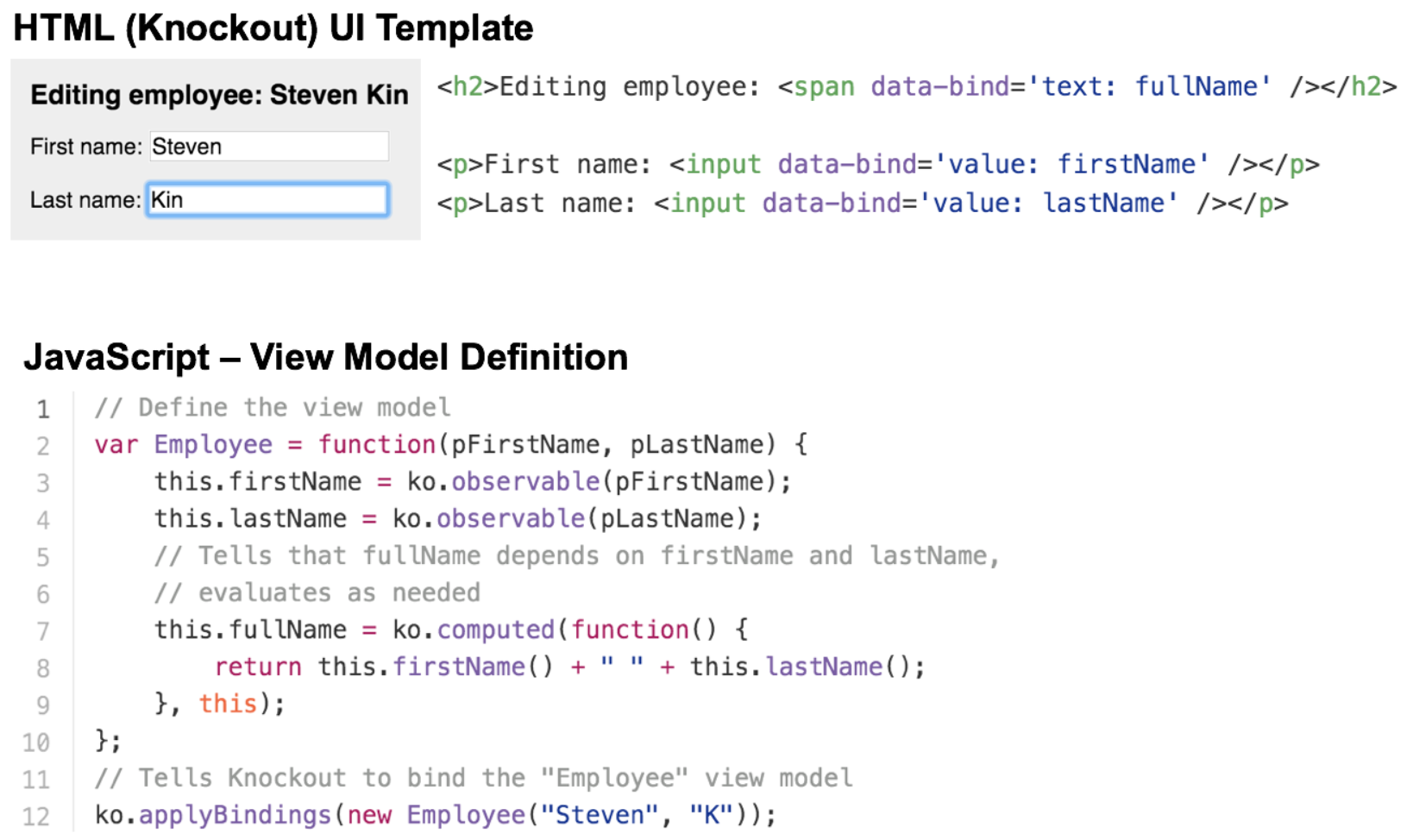
Listing 1: Basic example of Knockout's binding and computation feature
Every time a user changes the input value and tabs out of the field, the view model gets updated automatically. Notice the update of the fullName property: there is no need to catch “onchange” JavaScript events. Knockout knows when to update the UI. With Knockout, the HTML/JavaScript code keeps clean and maintainable.
RequireJS
When dealing with many JavaScript dependencies, which is typically the case for any serious web application, RequireJS helps to manage library references and lazy loading of resources. It does so by implementing the Asynchronous Module Definition (AMD) API. Instead of having multiple script tags in the HTML file, there is only one single line pointing to the require.js library. The following example shows a typical use case:

Listing 2: index.html: Asynchronous loading of required JavaScript files with RequireJS
The data-main attribute tells require.js to load js/main.js after require.js loads. The main.js file is responsible for loading all further JavaScript files (see Listing 3, below):
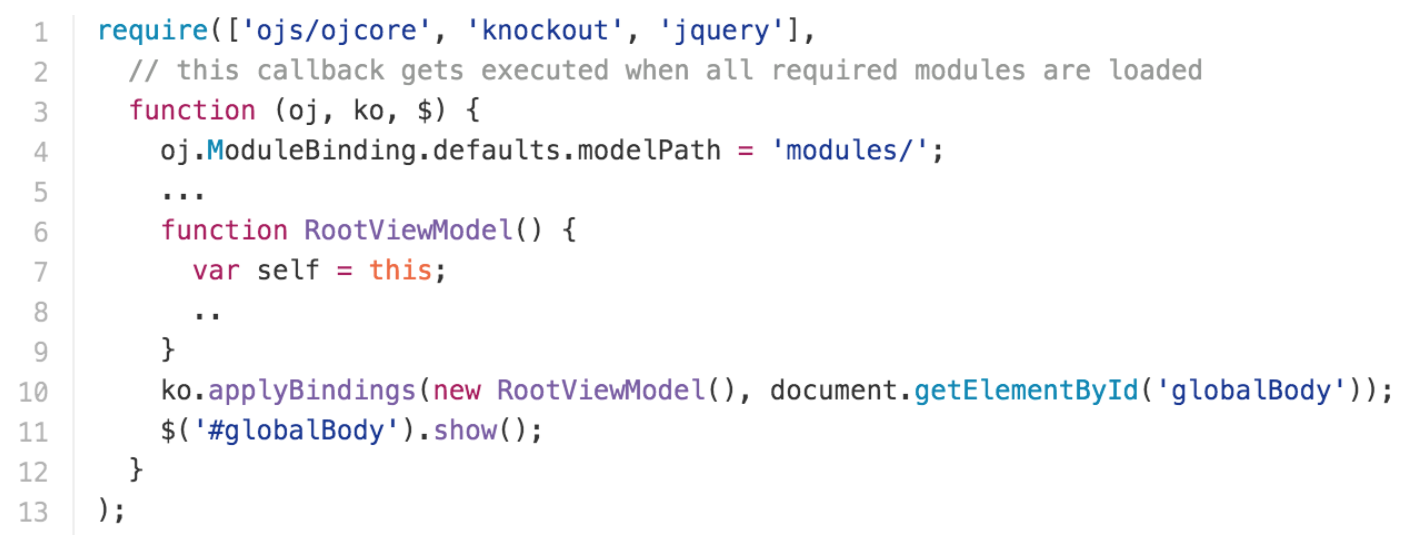
Listing 3: js/main.js: RequireJS loads required dependencies, then notifies via callback
Line 1 tells RequireJS to load three modules from the corresponding JavaScript files. The .js file extension is omitted. This convention makes it possible to upgrade for new module versions. It focuses on modules, not on the specific JavaScript files behind the scenes. Once the modules are loaded, the function in line 3 is called with references on each module if needed.
Note: In the JavaScript Module files, the define function will be used, not require--which is basically only for the initial bootstrap.
SASS
SASS (Syntactically Awesome Style Sheets) extends CSS3 and enables you to use variables, nested rules, mixins, and inline imports. Oracle JET uses the SCSS (Sassy CSS) syntax of SASS. Unless you want to create your own theme or override parts of the Alta theme, you do not really need to know many details about SASS. But it certainly will help you become an Oracle JET ninja.
Hammer.js
Oracle JET components use Hammer.js internally for gesture support. You do not need to know more about it to get started with JET development.
Rich UI Components Set
One of the most impressive things about Oracle JET is that it comes with a rich set of user interface components, including all traditional input elements for building beautiful UIs in great looking layouts, as well as comprehensive data visualization components. See Figure 3, below, to get a glimpse of some of the more complex UI components Oracle JET gives you.
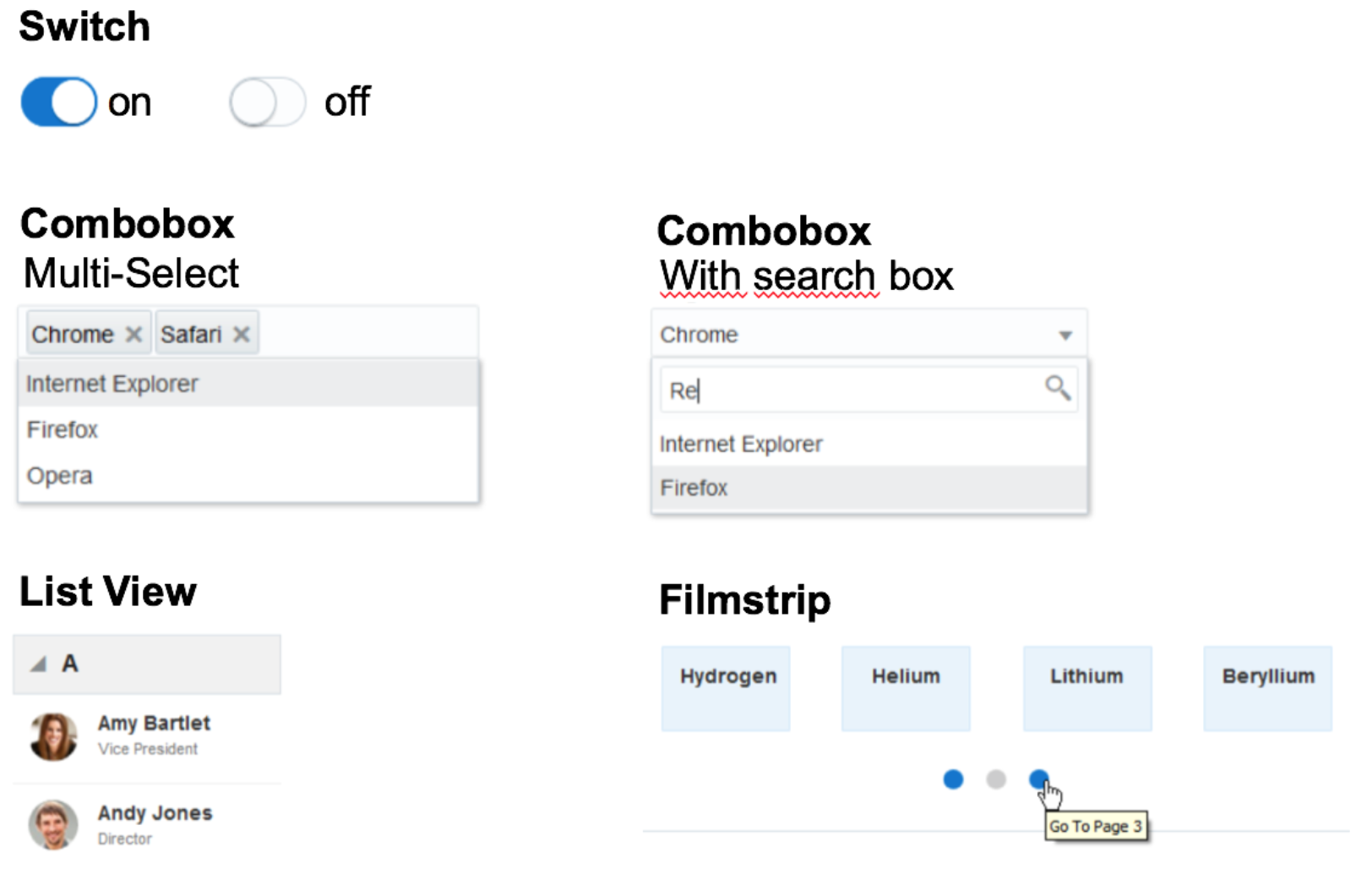
Figure 3: Modern user interaction components available out-of-the box
Beyond the UI component set, style classes are available for implementing a powerful 12-column responsive grid system that supports four different device sizes: small, medium, large, x-large. See the Oracle JET Development Guide for great examples.
Built with Accessibility in Mind
Accessibility does not get as much attention as it should. Accessibility means that someone working with assistive technology (like a screen reader) can explore and use the web application without a touch or mouse input device, using only keyboard and braille display. For the visually impaired, it is very important to have such color contrasts that the application is usable on black/white screens.
W3-Standard for Accessibility, the Web Content Accessibility Guidelines (WCAG) 2.0, and (since 2014) WAI-ARIA (Web Accessibility Initiative – Accessible Rich Internet Applications) 1.0 specifically address dynamic content and user interface components developed with Ajax, HTML(5) and JavaScript. They define semantics through additional attributes and metadata on HTML elements, making the web more accessible. Most screen readers nowadays are supporting these so-called WAI-ARIA attributes (e.g., role=“dialog“ on a DIV-element to tell the browser/screen reader that everything inside the DIV is meant to be content in a popup dialog).
Creating accessible web applications is a great challenge. Having a toolkit in which these requirements are implemented from the beginning is just awesome. Figure 4 shows an example of how Oracle JET implements the Input Date UI Component through proper usage of WAI-ARIA attributes:
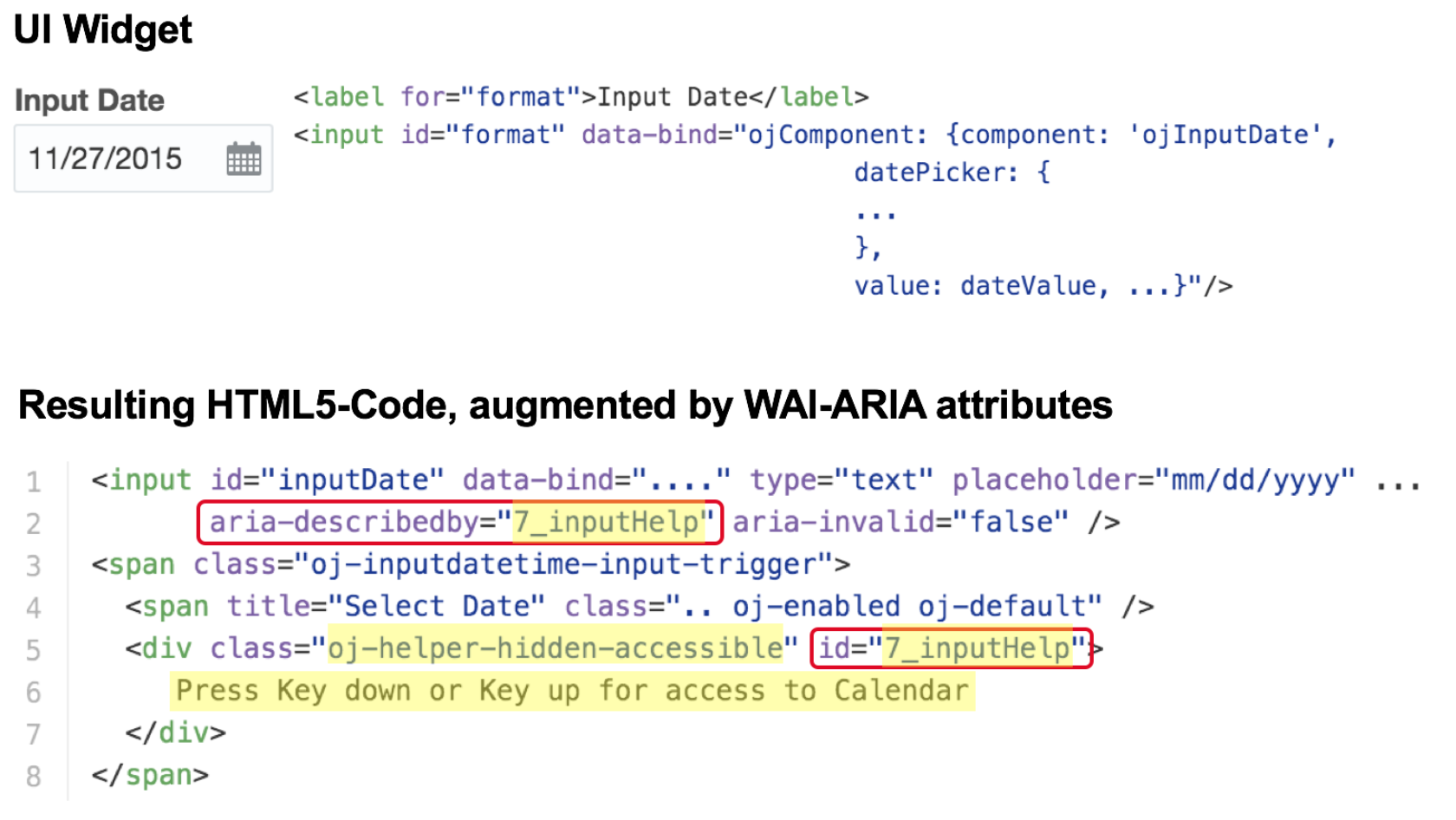
Figure 4: WAI-ARIA attributes make Oracle JET's InputDate (with DatePicker) UI component accessible by default
In the given example, the aria-describedby attribute is used in conjunction with a visually hidden div-element to give screen readers additional information on the input component. It is the resulting HTML5 code. There's no need to care about that when developing the UI with Oracle JET--the ojInputDate component handles all that.
Internationalization (i18n) and Localization (l10n)
Oracle JET has great support for i18n and l10n. For right-to-left languages (such as Arabic and Hebrew), there is built-in support in existing JET components (see example ojInputDateTime in Figure 5, below):
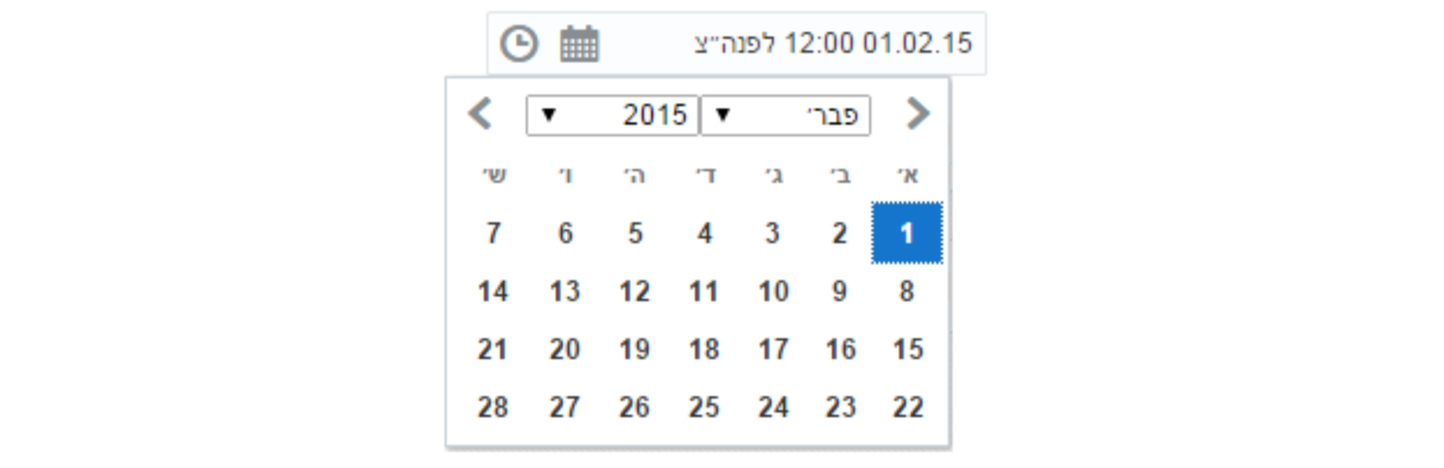
Figure 5: Internationalization with right-to-left language support
Creating custom resource bundles is also supported. Unlike in Java, the translations are not put into property files but into JavaScript files. To look up the defined translations, Oracle JET provides the oj.Translations object. See Listing 4, below, for an example of defining and using translations:
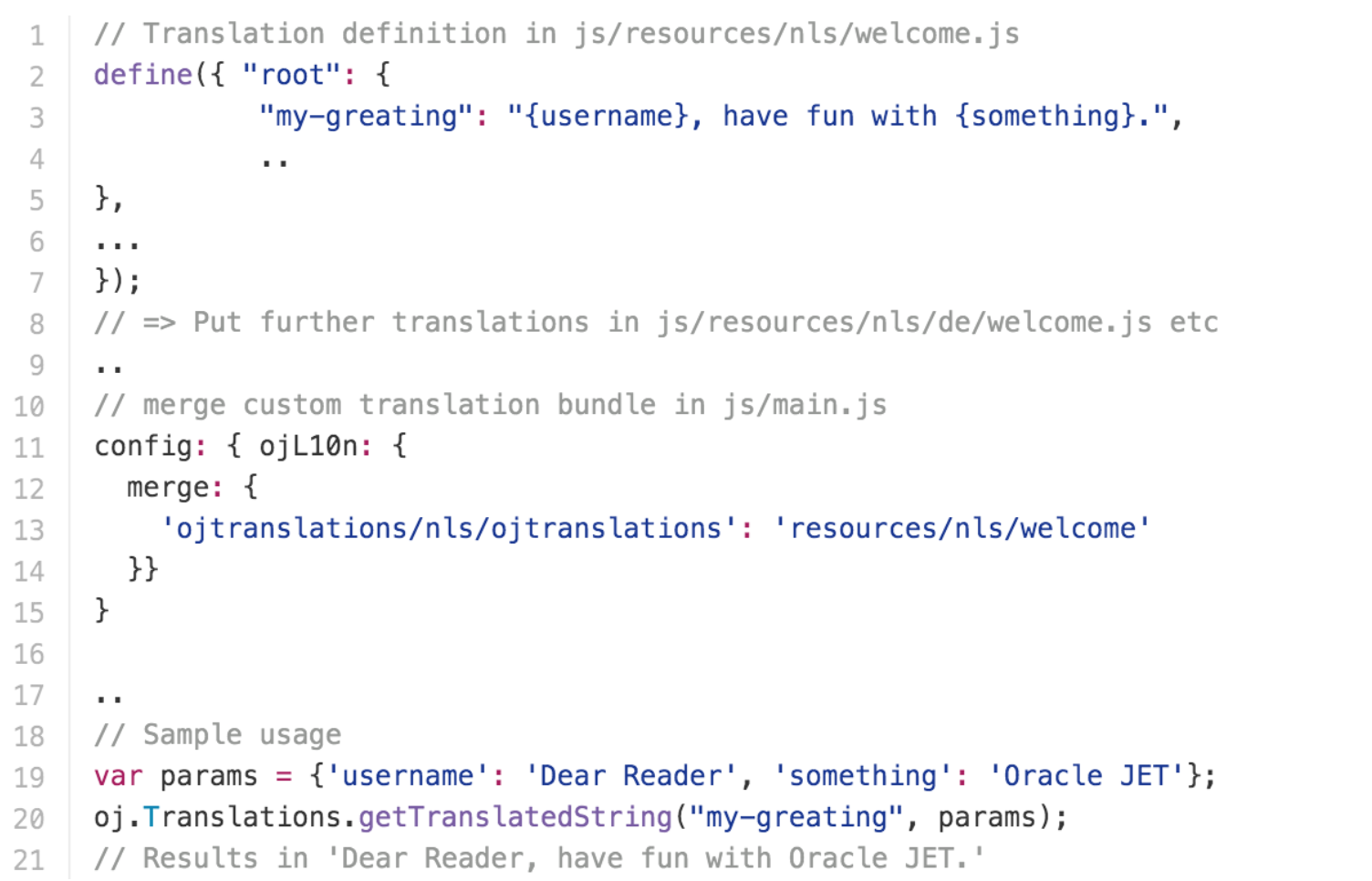
Listing 4: Defining and using translations in JavaScript file
Theming
The default theme in Oracle JET is Alta UI, implemented by utilizing SASS features. The only single 3rd-party CSS included is normalize.css. It is recommended that you extend the default Alta theme by overriding the desired parts or extending your own custom components. This makes total sense since the Oracle UX team has invested a lot of effort.
According to the Oracle JET development guide (v1.1.2), support for custom themes is also available.
Security
The most important part should be secured on your server-side. On the client side, you have to deal with some security aspects as well. Oracle JET does so by following common best practices inside its rich UI components. That is:
- Executing all JavaScript code in strict mode
- Not using inline script elements
- Not generating random numbers
- Escaping/sanitizing all generated HTML code
Oracle JET also brings an oj.OAuth API, which supports the OAuth 2.0 protocol, but I am not going into details about that in this article.
Finally, it is the developer's responsibility to follow current recommendations for writing secure JavaScript code (https://www.owasp.org/index.php/DOM_based_XSS_Prevention_Cheat_Sheet#Guidelines_for_Developing_Secure_Applications_Utilizing_JavaScript)
Working with the Oracle JET Cookbook
Developing web applications with Oracle JET may be different from the expected declarative approach. Instead, development is done pretty much by copying and pasting code from the Oracle JET Cookbook; it contains recipes for every UI component: the HTML5 templates code, the sample JavaScript code to handle events and setup the View Model, and a comprehensive API documentation. The most exiting thing about the JET Cookbook is that you can change the sample code and preview changes right inside the browser. Figure 6 shows the recipe for the slider component:
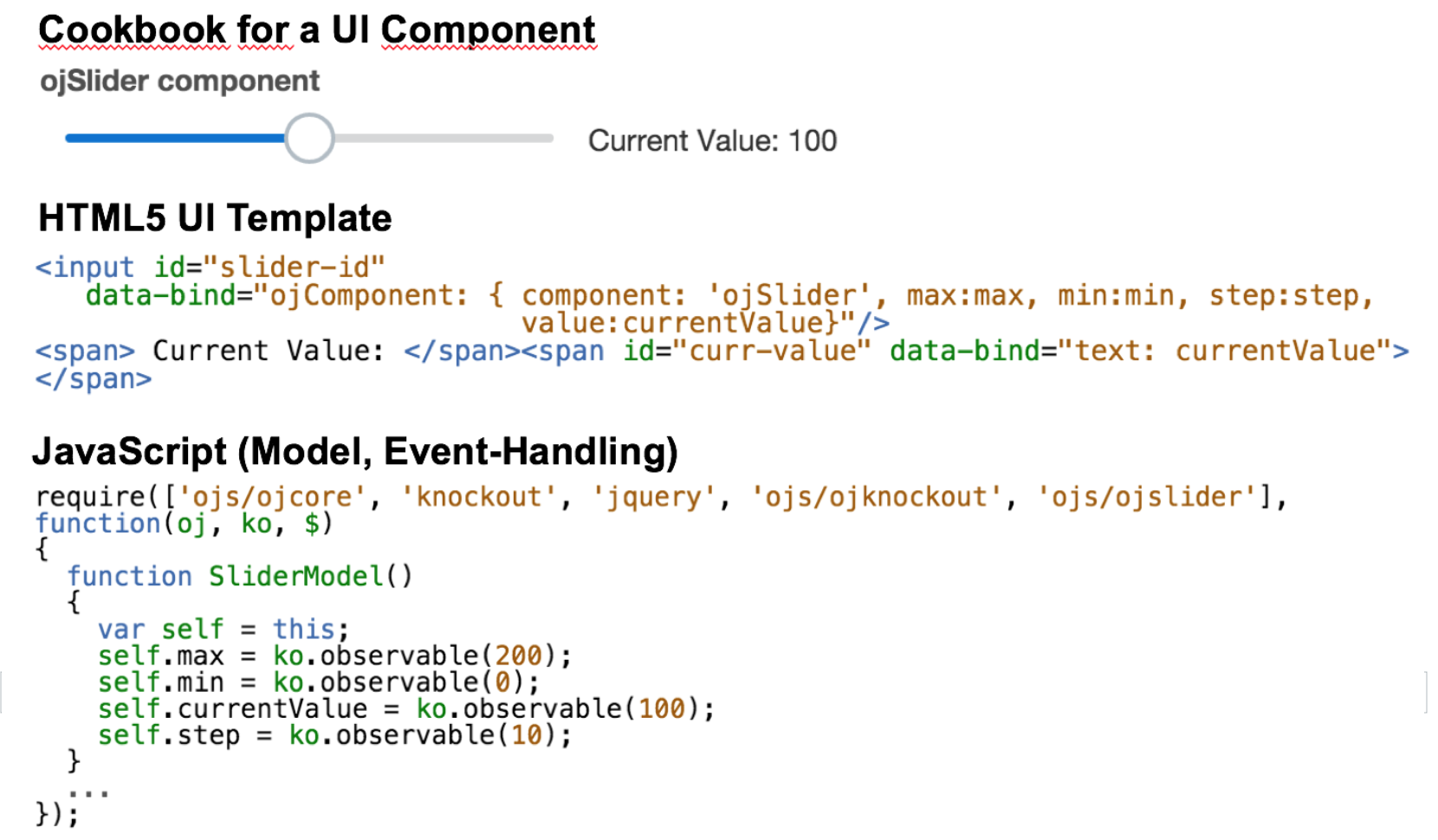
Figure 6: Oracle JET Cookbook recipes consist of a preview, a modifiable view template and corresponding JavaScript Event-Handler
Set Up a New Oracle JET Application
To actually start developing an Oracle JET web application, there is a QuickStart Basic Template ZIP file for download. The QuickStart Basic Template implements a single page application (SPA) that is structured for modular development using the described JavaScript libraries RequireJS and Knockout templates. See Figure 7 for the application source structure and the resulting preview:
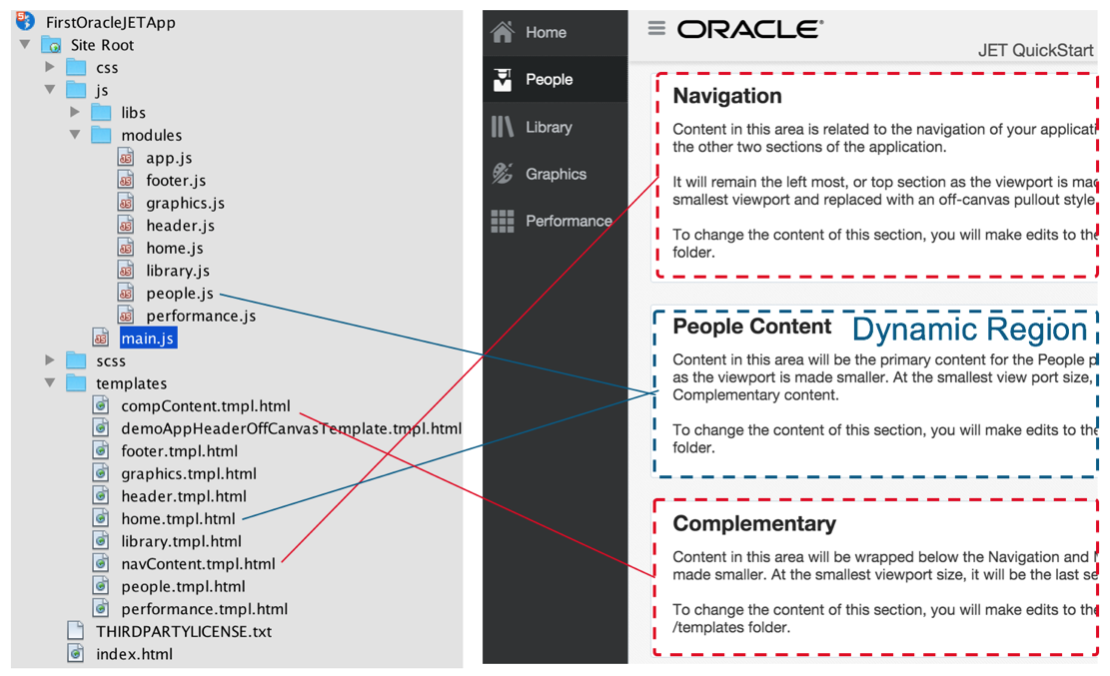
Figure 7: Modular Oracle JET Quickstart Template
Instead of having all the application markup in the index.html file, the template uses data-bind syntax and an ojModule binding to bind either a Knockout view template containing the HTML markup for the section or both the view template and the JavaScript file containing the ViewModel for any components defined in the section. See Listing 5:
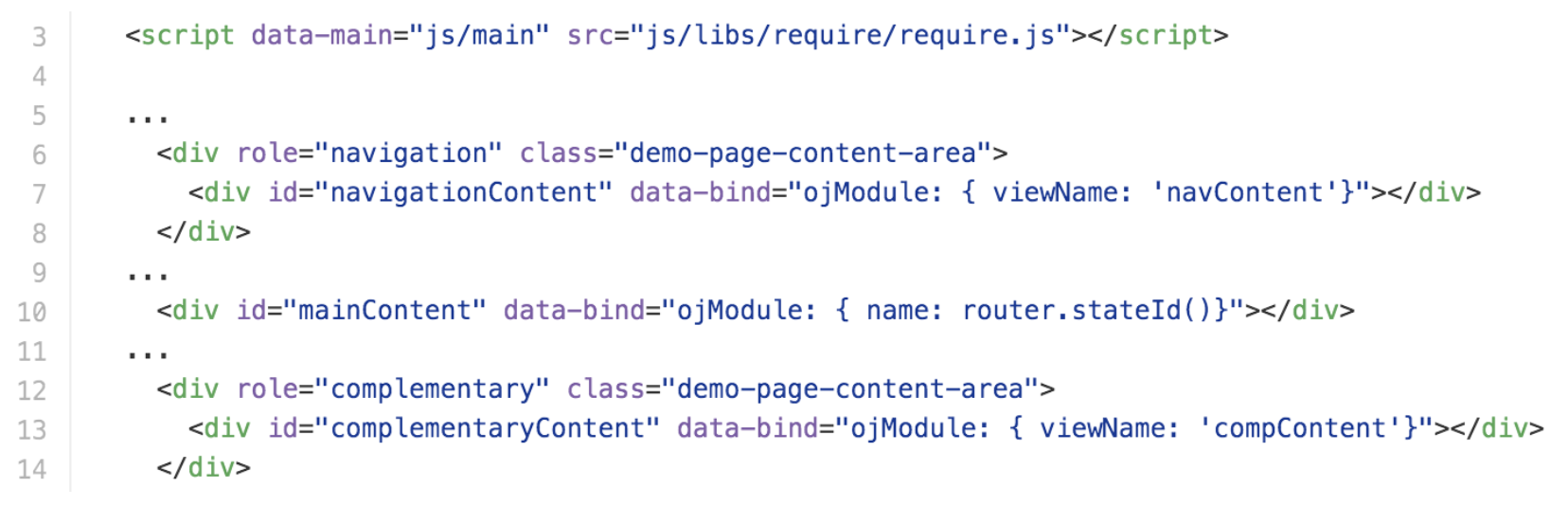
Listing 5: index.html: Modular SPA template
With the ojModule directive you are telling Oracle JET to bind the given div element to the navContent.tmpl.html (the suffix is predefined in main.js). This is how it works for the Navigation and Complementary regions. For the dynamic Main Content region, it derives the name (of the JS file that contains the ViewModel and the HTML template filename) from the router. The return value of router.stateId() is set to the current value of the ojRouter object. The ojRouter object is defined with an initial value of "home" in the application's main.js file, which bootstraps the application. See Listing 6:
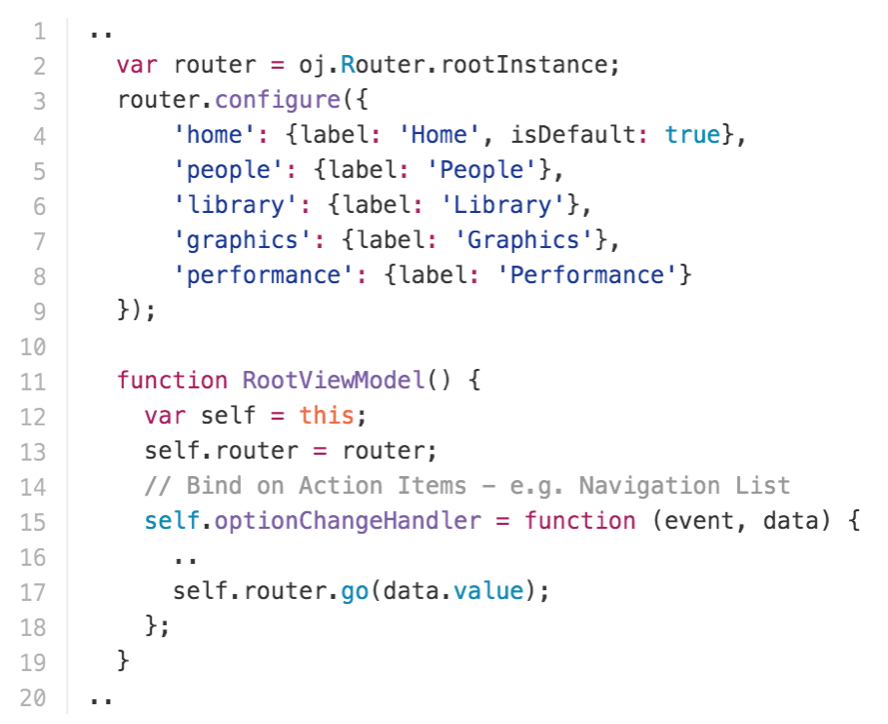
Listing 6: js/main.js: The ojRouter enables dynamic regions in Oracle JET
Since "home" is defined as default in the router configuration, Oracle JET will initially render home.tmpl.html template with the home.js view model.
Note: You will see „var self = this; self.firstName = etc…“ in nearly every view model. Since "this" refers to different objects depending on the call-stack / execution context, one can save a reference to a particular object by assigning it to another variable (here: "self“).
To summarize: the QuickStart template follows the Alta UI design principles and best practices and is a good starting point for new applications--just remove unneeded parts and you are good to go.
Going to Real World Requirements: Display Data from RESTful Services
To go beyond introductory basics, it is good to see a more sophisticated example. As described, it is common to consume data from RESTful services. Figure 8 demonstrates the result of the following basic example displaying a table of employees:
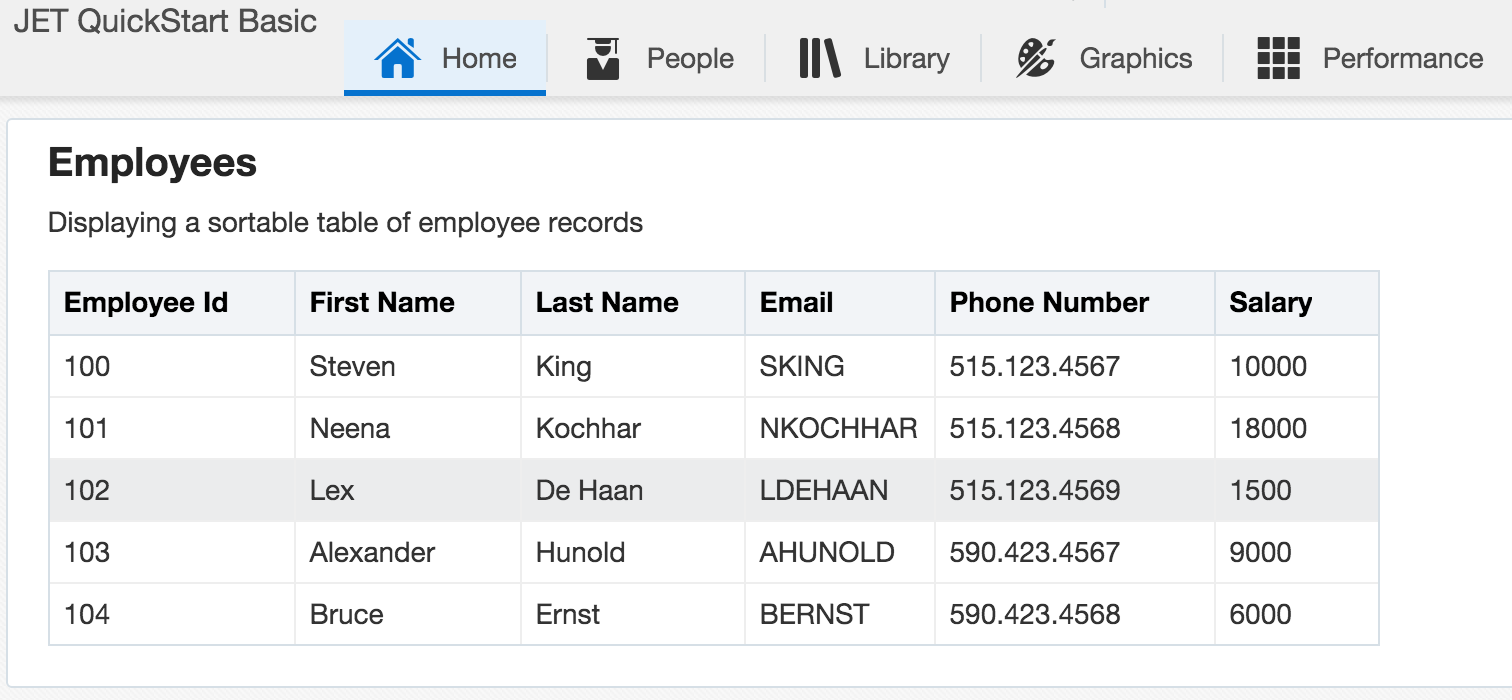
Figure 8: Displaying JSON data in a basic sortable table
As a newbie to Oracle JET, you need the following to create this by your own: JET Development Guide and JET Cookbook for the basic table, and of course a JSON source (e.g., https://gist.github.com/multikoop/98a664f3c39782a60913).
Starting with the HTML view, the template is pretty straightforward. Copy the code snippet and paste in home.tmpl.html (see Listing 7):
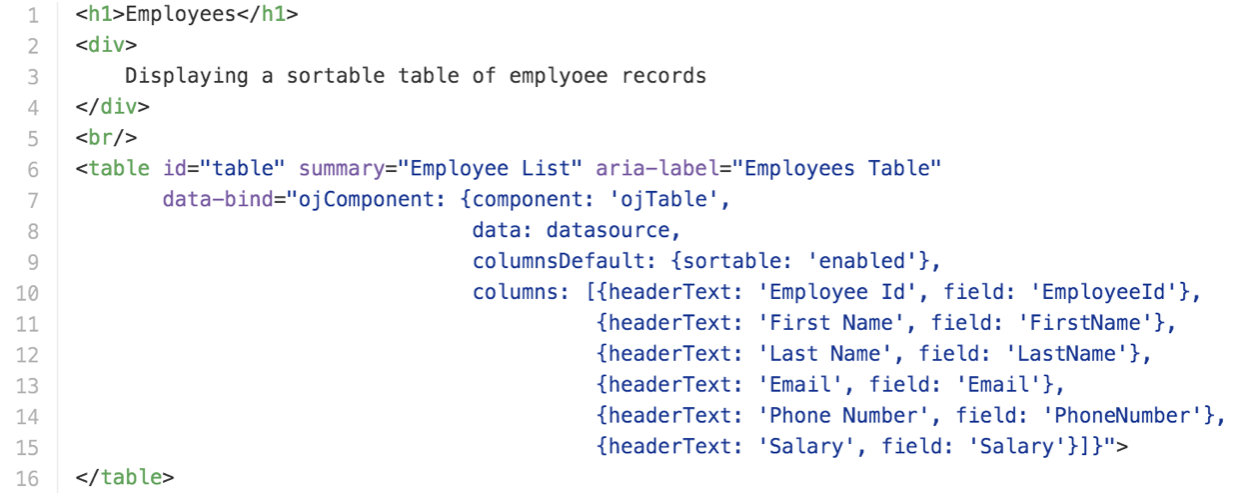
Listing 7: home.tmpl.html: HTML/Knockout template for a basic sortable table
Next, the bound datasource must be defined in the ViewModel. Actually, the datasource object is responsible for serving the Collection and fetching the JSON data. See Listing 8, below, for the empViewModel, defined in modules/home.js to get the idea.
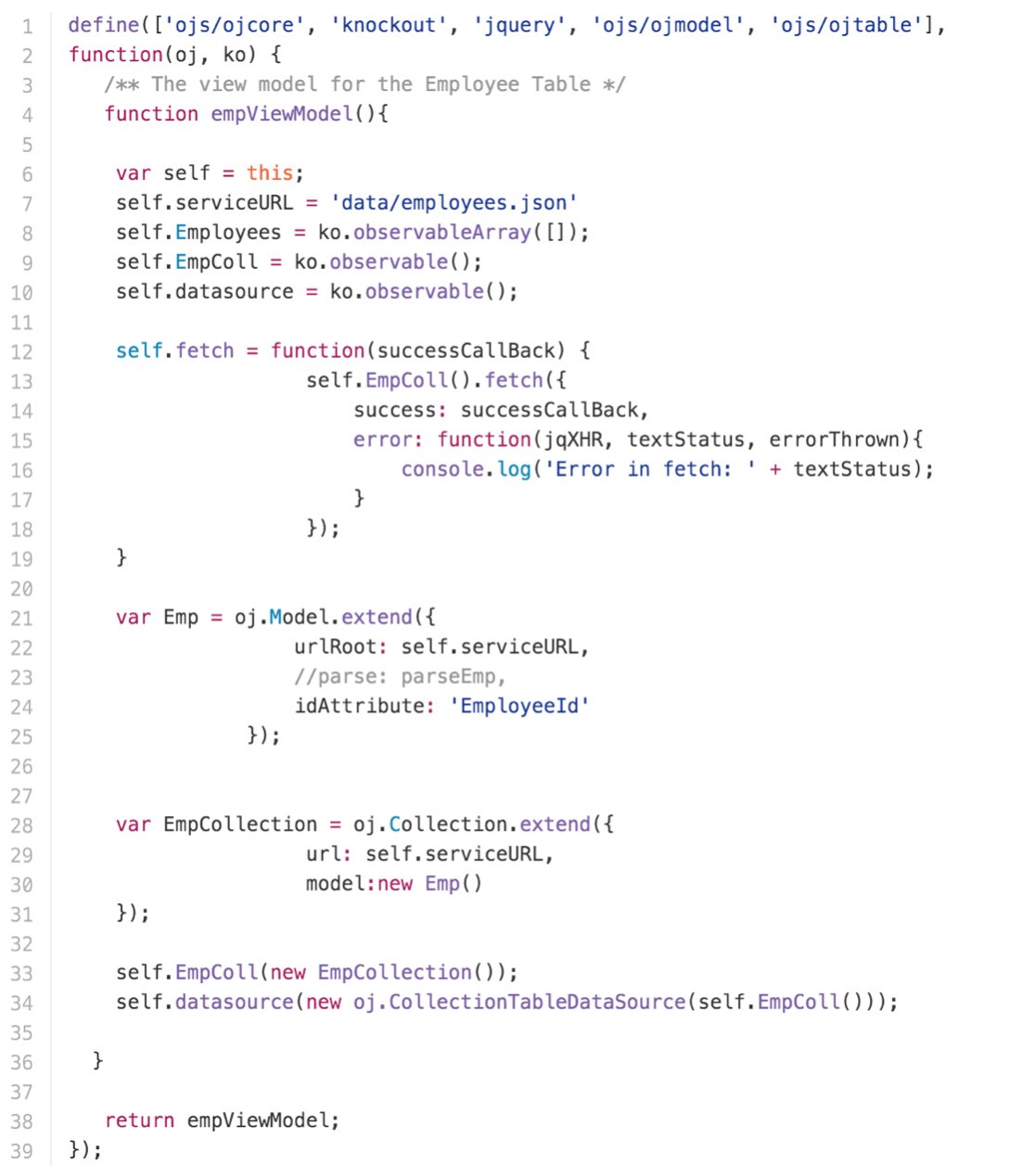
Listing 8: modules/home.js: The View Model for the employees basic table
The most interesting parts here are the concept of the Model and the Collection: Emp-Model defines a single record, while Collection represents a list of those records. Oracle JET brings some basics like the oj.Model, oj.Collection, and oj.CollectionTableDataSource, which triggers the underlying operations (fetch, parse, etc.) for you.
Optionally, if you need to customize the mapping between JSON attributes and your View Model, you can define a "Parse“ function. To keep the sample simple, it is commented.
Deploying and Running JET Applications
Oracle JET applications are typically just a collection of HTML, JavaScript and CSS files; you can just copy those files to any web server to serve the application.
In practice, you might want to bundle the Oracle JET application together with your backend RESTful services. Using JAX-RS you could bundle the application as WAR and deploy it to a Java EE application server (e.g., WebLogic Server). Another option could be to bundle it as a Node.js application if you want to stay in JavaScript land.
Tools and Best Practices
For lightweight HTML5/JavaScript development, there is pretty much no restriction in what development environment to use. Although you can go with Notepad, SublimeText, Atom, Brackets (with Brackets-Live feature), or Visual Studio Code, I have a recommendation for getting started.
NetBeans with Oracle JET Plugin
NetBeans has long had great support for HTML5, JavaScript, and even many JavaScript libraries and frameworks like Node.JS, Ember, Knockout, AngularJS, Backbone.js, etc. These built-in features make it particularly well-suited to be used for Oracle JET development.
Download NetBeans (minimum version 8.1), install and start it. Next, install the JET Plugin through Tools > Plugins > Search for JET and get going.
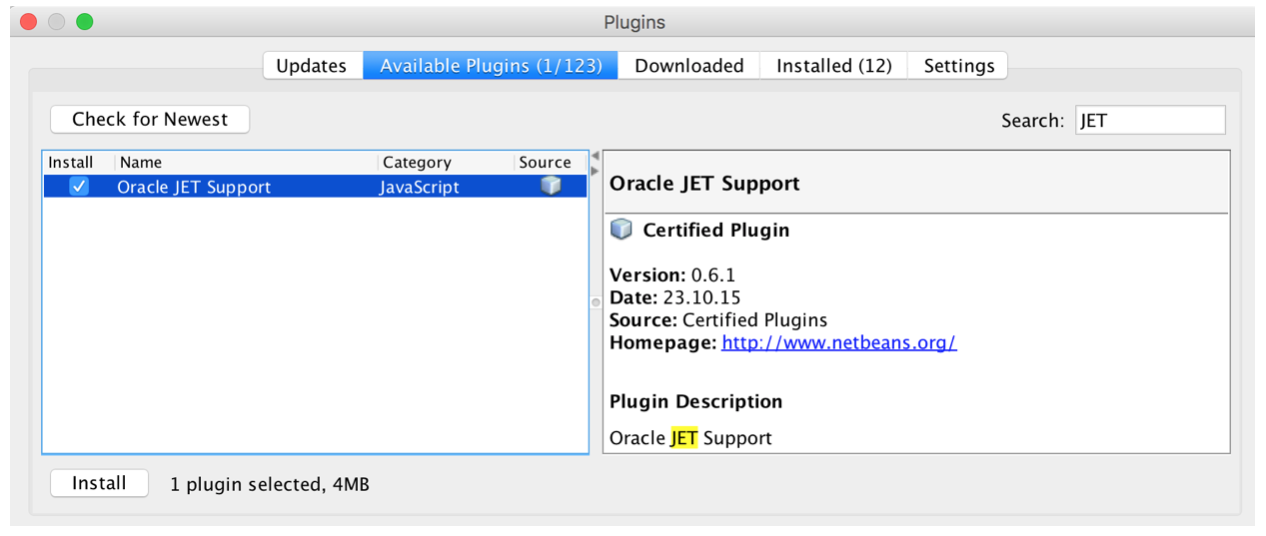
Fig. 9: Oracle JET Support Plugin for NetBeans
Once the Oracle JET Plugin is installed, select New Project and search for "jet“ to strip down the choice (See Figure 10):
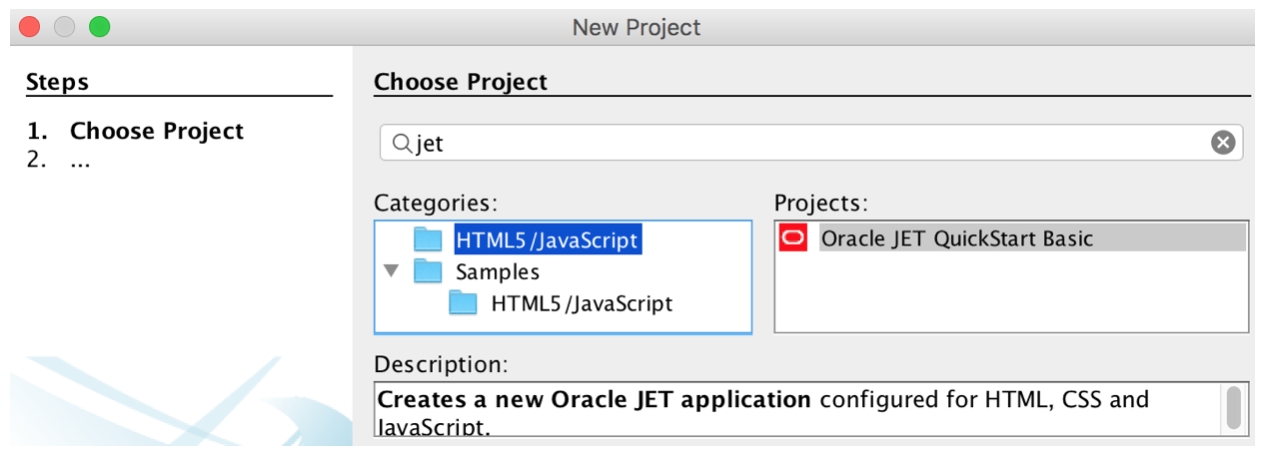
Fig. 10: Create a new project based on the Oracle JET QuickStart Template right from NetBeans
Lightweight web server and JavaScript debugging
To take full advantage of the NetBeans integrated tools for HTML5 development, you should install the NetBeans Connector extension for the Chrome browser (see https://netbeans.org/kb/docs/webclient/html5-gettingstarted.html for additional information). Once you've done that, it's breeze to make changes to your JET code and get a live preview in Chrome every time you save your changed files. You also get powerful capabilities for debugging JavaScript code. Figure 11 shows the main features of the NetBeans Connector for Chrome:
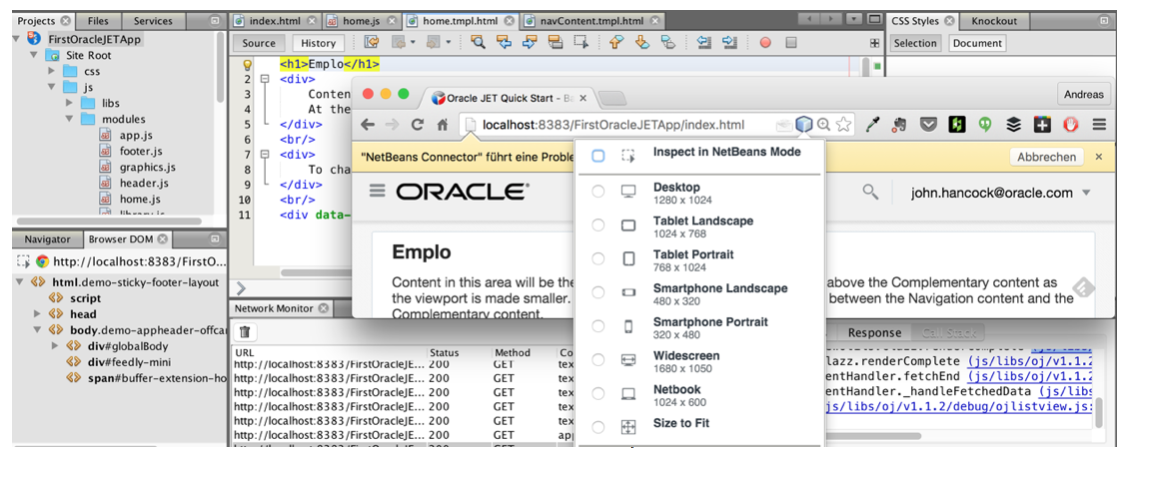
Figure 11: The NetBeans Connector greatly improves productivity while developing and debugging Oracle JET applications
Summary and a Look into the Future
Oracle JET provides a comprehensive toolkit in the ever-growing JavaScript landscape. Its complete set of UI components and Data Visualization Tools makes it very attractive. Beyond the visual features, it has great built-in accessibility support. Oracle JET uses proven open source JavaScript Libraries, making it easy to get started because there is a lot of learning material out there.
The built-in capabilities for theming, internationalization and security make it clear that Oracle JET is serious about the ongoing evolution of JavaScript for enterprise application development. Most of Oracle's Cloud Service UIs are built with JET right now.
Some parts are still missing to fully meet the "end-to-end“ requirement for full-stack client-side frameworks. In the JavaScript ecosystem, the build system Grunt/Gulp, Yeoman Generator, Jasmine for testing, etc., have become de facto standards. Having talked to the Oracle JET product managers, I expect that Oracle JET is going to adopt those build pipelines and that it will be open sourced on GitHub soon.
The development experience with Oracle JET is quite code-centric. With the upcoming Application Builder Cloud Service (ABCS)--which is built by JET--there will be a browser-based, declarative environment in which to build JET applications. Its main target audience will be business users/citizen developers, who will be able to build UIs by dragging and dropping. Extension hooks for CSS, HTML and JavaScript for implementation of more complex use cases are to be expected.
Do not forget: We are in JavaScript land. Parts of Oracle JET may change in future. Current trends are going towards more type save programming with TypeScript. The WebComponent Standardization is ongoing. The future of web application remains pretty exciting.
Further Information
Where to go from here? Start learning JavaScript. Download NetBeans and install the Oracle JET Plugin through the Plugin Manager. It’s the easiest way to get started. Move on with the Oracle JET Development Guide for technical details.
Stay informed of Oracle JET product updates and community driven content by following:
Have fun!
Resources
About the Author
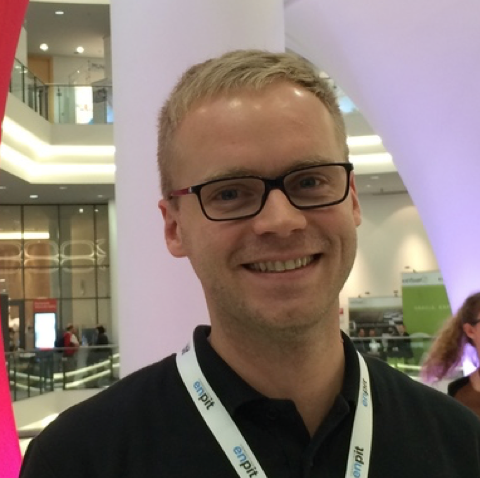
Oracle ACE Director Andreas Koop (@andreaskoop) is working as Solution Architect, Technical Lead or Trainer on different IT projects using Oracle technology. He is co-founder of enpit, a German-based company specializing in Consulting Services for Oracle Middleware technologies and Oracle Cloud offerings. He enables customers to successfully implement Business Solutions based on Oracle Middleware & Oracle Cloud products. As an active member of the German Oracle User Group (DOAG) and regular speaker at different conferences, Andreas shares his knowledge with the worldwide Oracle Community on his blog, in community meetings, on GitHub or on social media channels. In his spare time he enjoys life with his wife, family and friends. Whenever time permits, Andreas is making music, playing soccer, and biking.
This article represents the expertise, findings, and opinion of the author. It has been published by Oracle in this space as part of a larger effort to encourage the exchange of such information within this Community, and to promote evaluation and commentary by peers. This article has not been reviewed by the relevant Oracle product team for compliance with Oracle's standards and practices, and its publication should not be interpreted as an endorsement by Oracle of the statements expressed therein.