by César Hernández
Learn how to take advantage of Maven to automate the development lifecycle and the management of Java dependencies.
Maven is a powerful build automation tool mature enough to be nowadays the de facto tool for dependency management in Java projects. It is also a crucial part of the principle of continuous integration in the Java ecosystem because of its automation, build, package, and distribution mechanisms and capabilities.
One of the key components of the Maven tools is repositories—places where you can obtain and share your dependencies (artifacts). These repositories allow to developers to access thousands of tools, libraries, frameworks, and so on from the open source community and from leaders of the IT industry. In early 2015, Oracle launched the Oracle Maven Repository to facilitate development work inside and outside the Oracle Fusion Middleware ecosystem.
This article aims to help you build a solid foundation about how Maven works and how you can take advantage of this powerful tool to manage your Java dependencies in an automated manner.
Dependency Management
As you develop applications that require Java APIs that extend the base functionality of the language, you might have the need to import libraries (commonly, as files that have the .jar
extension) developed by third parties. It is at this point that your applications start to have dependencies.
The process of adding, updating, deleting, and distribute such dependencies among the members of a development team is complex and highly error-prone. A first approach that might be intended to reduce complexity for such dependencies is using versioning in a repository such as Apache Subversion or GIT. Although this approach is practical for the source code, it is not practical for dependencies, because it requires additional manipulations, such as the resolution of transitive dependencies that are produced when a dependency you import into your project needs one or more other libraries.
Through standardization and automation, and by following the principle of convention over configuration, Maven provides mechanisms that help resolve conflicts that arise when there are transitive dependencies that have different version numbers.
Standardizing Maven-Based Projects
Maven is based on the concept of a project object model (POM), which allows the objects (dependencies, project metadata, and plugins) to be explicitly declared to standardize the construction and packaging of Java projects that together form an application or system. The Maven configuration file used for this purpose is named pom.xml
.
For practical purposes, the basic structure of a pom.xml
file can divided into three sections:
1. General information about the project. This section contains information that is unique to the artifact that the project generates. The following are the mandatory fields:
groupId
: Identifies the group to which the project belongs
id
: Specifies the project name
packaging
: Specifies the package type to be produced by Maven (JAR or WAR)
2. Dependencies section. This section lists the dependencies that the project uses. At this point, you can even indicate the scope of each dependency. For example, you can indicate that the JUnit dependency is going to be taken into count only for unit testing purposes but not will be included as part of the project's final packaged artifact.
3. Plugins section. This section contains the declaration of plugins that extend the basic functionality of Maven. This feature has become very popular because Maven officially provides support to over 50 plugins, and the list of plugins developed by third parties is long. There are plugins for packaging, reporting, static code analysis, integration testing, and so on.
Installing Maven
The binary distribution is available from the official site. After it is downloaded, it must be decompressed. Then you must add an environment variable in your operating system point to the bin
folder inside the folder structure created by the decompression process.
To check the installation, run the following command at the command line:
mvn -version
Figure 1 shows the message that is displayed to indicate a successful installation:

Figure 1. Output indicating a successful installation
Maven Command Syntax
Here is the syntax:
mvn [_options_] [_goal(s)_] [_phase(s_)]
where:
mvn
: This is the command you use to run Maven.
[_goal(s)_]
: A goal represents a Maven plugin that you can add to a stage to specify or extend the behavior of that particular phase.
[_phase(s)_]
: A phase indicates a specific phase executed for the construction of the project.
To see the entire list of goals and phases, see the official documentation.
Creating a Java Project with Maven
To create a Java project with Maven, you must indicate the type of action you want to execute (_goal_
), identify unequivocally your project (groupId
and artifacId
), and select the initial template from which you want your project be created (archetypeArtifacId
).
In the following example, for practical purposes, Maven's console interaction mode (interactiveMode
), which can be used to provide additional information, is disabled:
mvn archetype:generate -DgroupId=com.yourorganization -DartifactId=my-application1 -DarchetypeArtifacId=maven-archetype-quickstart -DinteractiveMode=false
Figure 2 shows the standard Maven structure provided by the maven-archetype-quickstart
archetype:
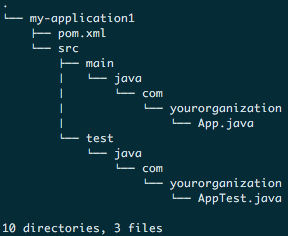
Figure 2. Standard Maven structure
Within the obtained directory structure, notice that Maven also creates classes and Java packages as well as the pom.xml
file (see Figure 3).
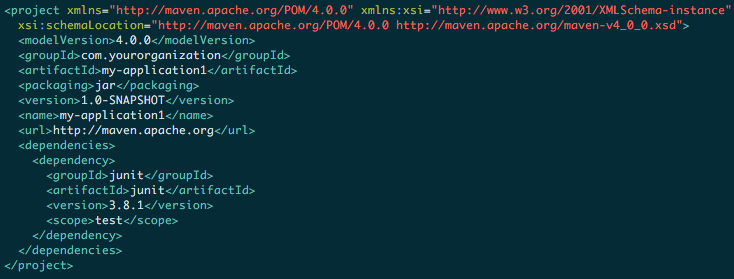
Figure 3. The pom.xml
file
Inside the pom.xml
file, the version of your project is automatically filled with 1.0-SNAPSHOT
. The snapshot version is used to denote that your project is under development and subject to constant changes. As good practice, after the initial development phase ends, you should update the version of your project to a specific version that does not include the word SNAPSHOT. This change is done simply by editing the pom.xm
l file. It is also worthwhile to indicate that the packaging of the project will be a JAR artifact.
Automating the Development Phases of a Java Project Based in Maven
Maven uses a construction lifecycle to automate various stages of your project: compilation, unit testing, packaging, integration testing, additional verification, and distribution of the resulting package to a local Maven repository and/or a centralized repository. When you install Maven, it automatically creates a local repository for you (usually in a folder named .m2
), which serves as a point of cache between the Maven internet servers and your workstation.
Inside the directory my-application1
, you can run multiple stages of your project in an independent manner by using the following commands.
mvn compile
This command compiles the project and creates a new directory called target
where your compiled classes are stored. It is important to note that at this stage, Maven has not yet carried out the packaging stage (the JAR file generation).
Figure 4 shows the generated structure:
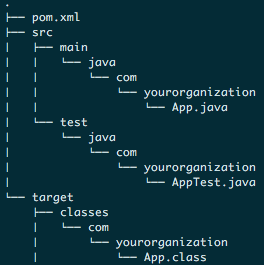
Figure 4. Structure created during the compilation phase
mvn test
This command compiles the project and then runs the unit test phase of the project (see Figure 5).
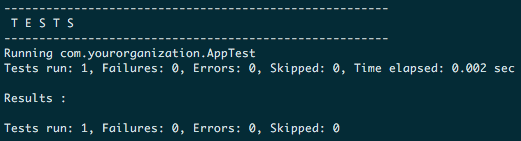
Figure 5. Unit test phase
mvn package
This command compiles the project, runs the unit testing phase, and finally packages the project into a JAR file. The generated JAR file is automatically stored within the target
folder (see Figure 6).

Figure 6. Package phase
mvn install
This command compiles the project, runs the unit testing phase, packages the project into a JAR file, and finally installs a copy of the JAR file into the local Maven repository. Remember that the local repository is the .m2
folder located in your home
directory on your workstation (see Figure 7).
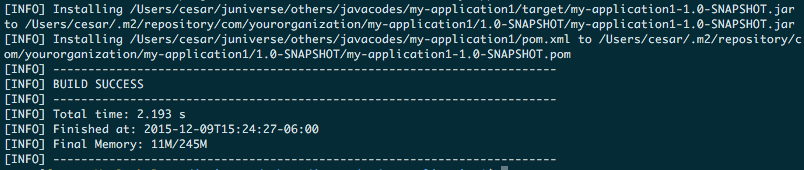
Figure 7. Installation phase
As shown in Figure 7, the folder called .m2
has been modified to add a copy of the artifact (the JAR file) that was generated.
Integrating Development Environments with Maven
Currently Maven has extensive integration with popular integrated development environments (IDEs) for Java development. This, along with continuous integration and continuous delivery, offers a great synergy to speed up your software application lifecycle.
Maven Repositories
The default Maven repository on the internet is Maven Central. Many other repositories are available, depending on an organization's needs and software licenses policies.
The release of the Oracle Maven Repository offers developers a number of dependencies and plugins that will further facilitate the development of Java projects in different areas (Java ME, Java SE, Java EE, and JavaFX) inside and outside the Oracle Fusion Middleware ecosystem.
Some of the advantages can be easily seen. For example, in the WebLogic Development Maven Plugin allows you to manage Oracle WebLogic Server by doing tasks such as installation, creation of Oracle WebLogic Server domains, and starting, stopping, and deploying applications and modules.
Maven Plugins
One key factor that had allowed Maven stay up to date with emerging technologies is its plugin capability. These capabilities range from old-school duties, such as adding a software license to every Java class of a project, to the creation and management of a Docker container lifecycle. The Maven site provides the official list of well-known Maven plugins.
The Power of Maven Artifacts
In simple words, Maven artifacts are project templates that can include a predefined folder structure, configuration files, Java classes, and a pom.xml
file with dependencies, plugins, and configurations.
In one simple command you can obtain a ready-to-deploy JAX-RS web service or an Enterprise JavaBeans (EJB) module. The Oracle WebLogic Server Maven archetype covers creating a wide range of projects, such as a Basic WebApp project, a WebApp with EJB project, a Basic MDB project, and a Basic WebServices project.
Conclusion
Maven allows you to optimize the development time for systems composed of Java projects by providing a standardized way to work and automate various stages during the construction, validation, packaging, and distribution of such projects. The next time you use Maven, remember the basic concepts covered in this article to smooth your user experience.
See Also
About the Author
César Hernández is a software architect who has been working with Java since 2008. He is an Oracle Certified Professional and holds a master's degree in IT management. His experience in the design, development, testing, and performance tuning of enterprise Java applications has led him to be a member of OTN Speaker Bureau, an active speaker in Latin America Java User groups (JUGs) and OTN tours, a Guatemala Java community leader, a cofounder of the JEspañol initiative, and the founder of the tFactory open source tool.
Join the Conversation
Join the Java community conversation on Facebook, Twitter, and the Oracle Java Blog!